Mastering iOS Deep Linking and Universal Linking: A Comprehensive Guide
Oct 17, 2024
App users expect seamless navigation, both within the app and from external sources like web pages or emails. Two major techniques to achieve this in iOS are Deep Linking and Universal Linking. This guide covers the essentials, including what they are, how to implement them, code snippets, FAQs, and third-party services to streamline the process.
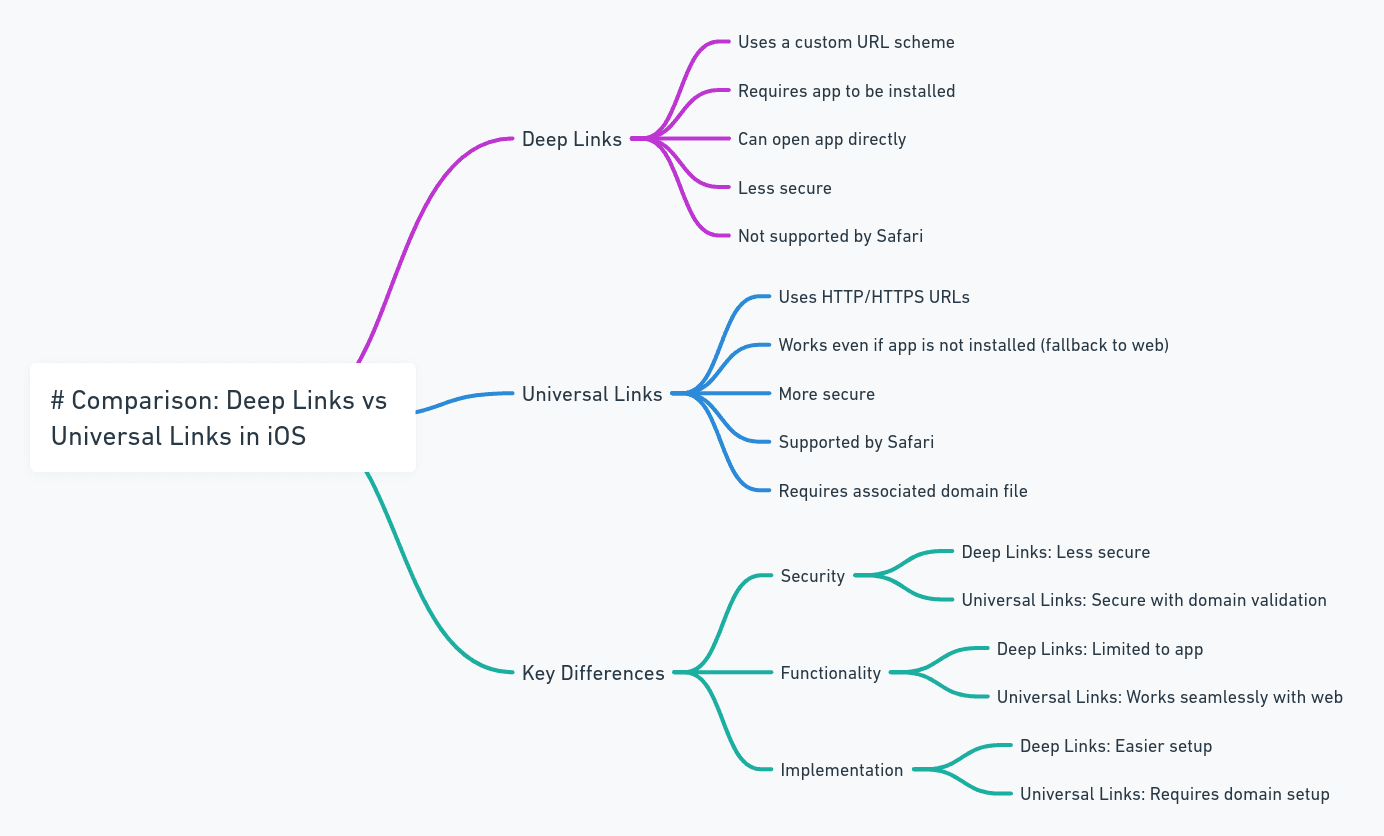
What are Deep Links and Universal Links?
Deep Links in iOS
Deep Linking refers to a URL that brings users directly to specific content within an app, bypassing the app’s home screen. With deep linking, users can be navigated directly to the relevant content, enhancing user experience and engagement.
However, traditional deep links have limitations:
They only work if the app is installed. If not, users encounter a broken link.
For non-installed apps, deferred deep linking attempts to solve this issue, but it can be tricky.
Universal Links in iOS
Apple introduced Universal Links in iOS 9 to improve deep linking. Universal links:
Allow users to open content in the app if it’s installed or fall back to the website if not.
Are secure, as they require ownership of the domain.
Prevent broken links by defaulting to web content when the app is not installed.
Universal links are recommended for most iOS deep-linking needs due to their flexibility and user experience benefits.
Implementing iOS Deep Links
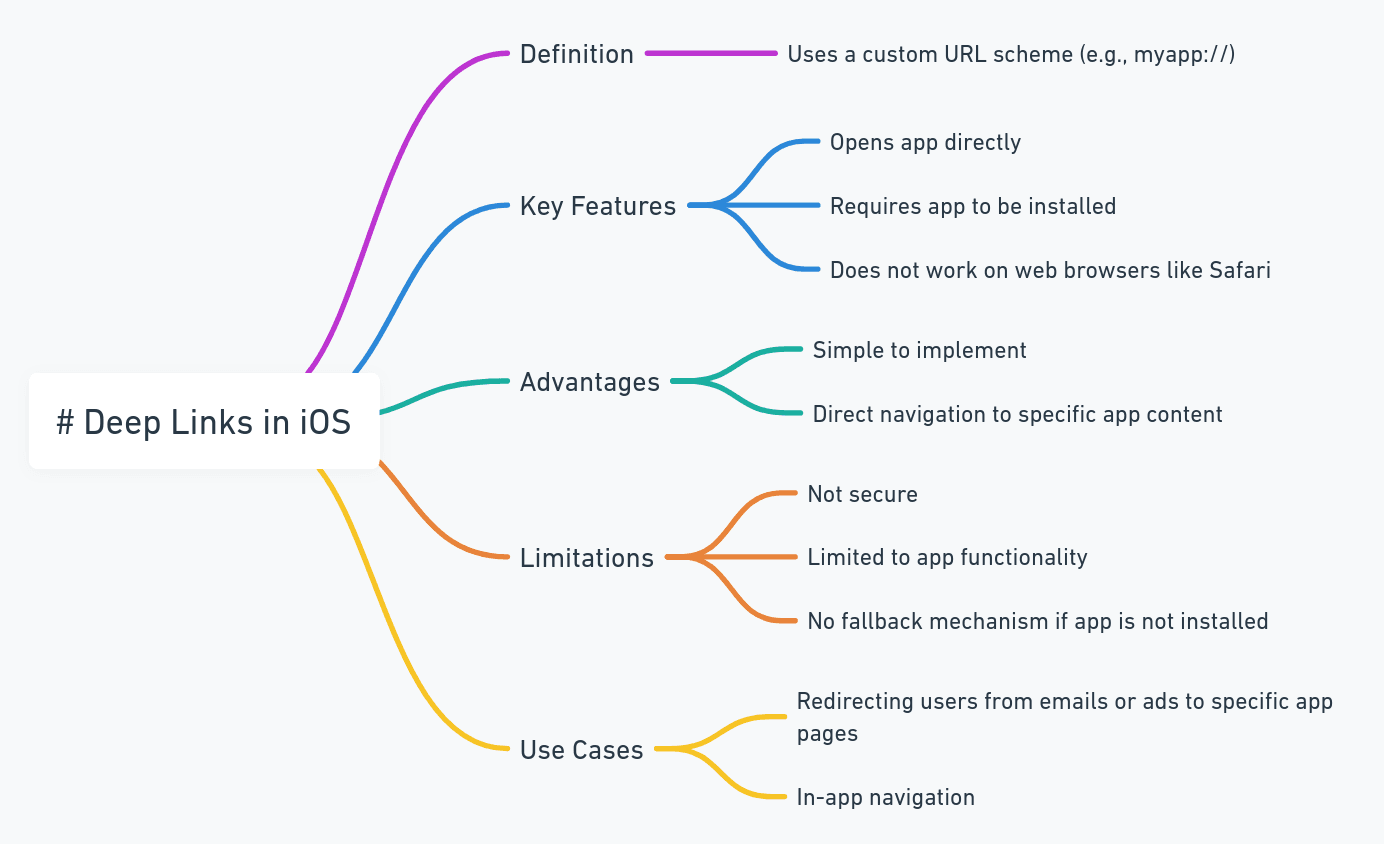
1. Traditional Deep Linking Setup
For traditional deep linking, we use URL schemes, which are custom URL structures that launch the app and navigate to specific pages.
1. Define a Custom URL Scheme:
In Xcode, open your project.
Go to Info and add a new URL Types entry.
Enter a unique URL Scheme, e.g., myapp.
2. Handle Deep Links in App Delegate:
Implement the application(_:open:options:) method in your AppDelegate.swift to handle the incoming URL.
With this setup, URLs like myapp://page1 will directly open the app and take users to Page1. However, these URLs only work if the app is already installed, which brings us to Universal Links.
Implementing Universal Links in iOS
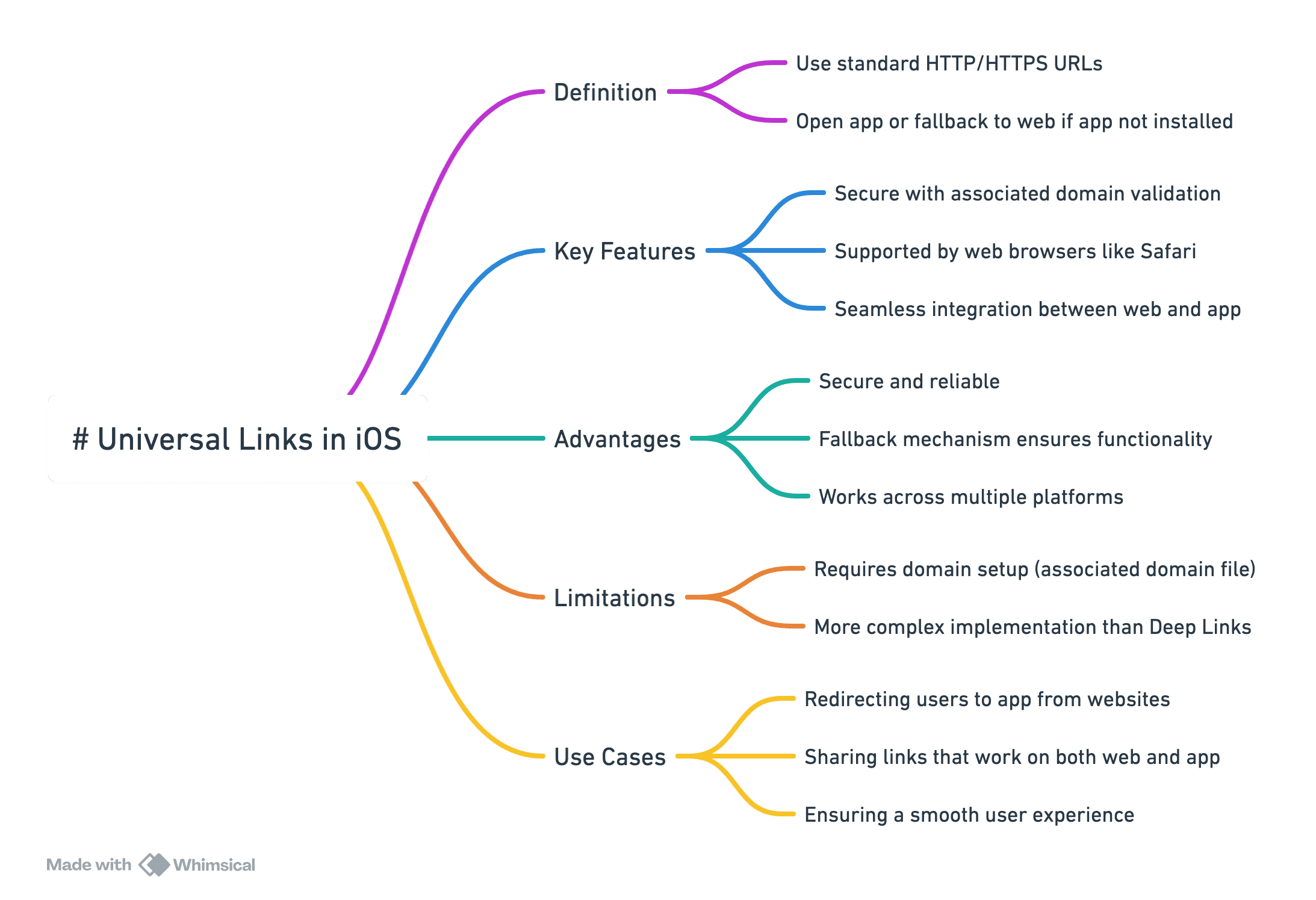
1. Setting Up Apple App Site Association (AASA) File
Universal Links require the Apple App Site Association (AASA) file, hosted on your website.
1. Create the AASA file:
The apple-app-site-association file is a JSON file that specifies the paths in your domain that should open in your app.
2. Configure Associated Domains in Xcode:
Go to your project settings, select your target, and go to Signing & Capabilities.
Add the Associated Domains capability.
Enter your domain prefixed with applinks:, like so:
3. Handle Universal Links in App Delegate:
Use application(_:continue:restorationHandler:) to handle universal link navigation.
Testing Your Universal Links
Install the app and try clicking the universal link on your iOS device.
If the link redirects to the web, ensure your AASA file is correctly configured and served over HTTPS.
You can also use Apple’s Universal Links Validator to verify that your setup works.
Certainly! Here’s an updated section with links to each of the third-party services mentioned for iOS deep linking and universal linking.
Third-Party Services for Deep Linking and Universal Linking
Managing deep links and universal links can be complex, especially for large-scale apps with many links to maintain. Several third-party services provide comprehensive solutions for deep linking, universal linking, and deferred deep linking.
1. Branch.io
Branch is a popular deep linking solution that handles both deferred deep linking and universal linking. Key features include:
Support for cross-platform linking.
Powerful analytics on link clicks and installs.
Deferred deep linking, allowing users to be taken to specific content after app install.
Branch simplifies setting up links for iOS and Android with advanced tracking and attribution.
Firebase Dynamic Links by Google offers a robust solution for creating smart links for both iOS and Android. Key features include:
Auto-detection of platform (iOS, Android, or web).
Deferred deep linking.
Integration with other Firebase tools like Analytics and Cloud Messaging.
Firebase Dynamic Links is free and integrates well with Firebase’s ecosystem for tracking and notifications.
3. Adjust’s Deep Linking Solutions
Adjust provides deep linking as part of its app measurement platform, focusing on mobile marketing. Features include:
Deferred deep linking and re-engagement.
User-level analytics.
Customizable link routing based on device, user behavior, or campaign.
Adjust’s deep linking works well for apps focused on marketing and retargeting.
AppsFlyer’s OneLink service specializes in marketing attribution and allows for deep linking across platforms. Notable features include:
Multi-platform deep linking with user segmentation.
Deferred deep linking and custom routing.
Rich analytics for user acquisition and campaign performance.
AppsFlyer is ideal for marketers who need insights into link performance and user behavior.
5. Kochava
Kochava’s deep linking platform focuses on user engagement and marketing. Key features include:
Cross-platform deep links and universal links.
Deferred deep linking with user tracking.
Integrations with other marketing and analytics tools.
Kochava is suited for companies needing precise attribution and deep linking for marketing purposes.
Each of these services offers robust capabilities for handling deep linking, deferred deep linking, and universal linking, making it easier to guide users through seamless navigation experiences across platforms.
Frequently Asked Questions
1. What’s the difference between deep links and universal links?
Deep links are app-specific URLs that open the app but fail if the app isn’t installed. Universal links, however, have fallback functionality, directing users to the website if the app isn’t installed.
2. Why doesn’t my universal link open my app?
Common reasons include:
The AASA file isn’t configured correctly.
The AASA file isn’t served over HTTPS.
The Associated Domain capability isn’t added in Xcode.
3. How can I prevent universal links from opening in Safari?
Ensure the AASA file is correct, as an improperly set-up AASA file leads to links opening in Safari.
4. Do universal links work on older iOS versions?
Universal links work on iOS 9 and later. On older versions, you’ll need to rely on traditional deep links.
5. Can I use a single link for both Android and iOS?
Yes, but you need to handle it appropriately. Tools like Branch and Firebase Dynamic Links can generate a single link that redirects to the App Store or Google Play if the app isn’t installed.
6. How can I handle universal links when my app is updated?
Universal links continue to work across app updates as long as the domain and AASA configuration remain unchanged.
7. Can universal links handle deferred deep linking?
Yes, some third-party services (listed below) offer deferred deep linking using universal links, which can redirect users to specific content after they install the app.